Hello there,
As I am working on a project in Godot and I’m facing a bit of a challenge regarding implementing smooth camera movement. I’ve tried a few approaches, but none seem to give me the fluidity I’m aiming for.
My goal is to have the camera smoothly follow the player character as they move around the scene. I’ve experimented with using the “look_at” function and adjusting the camera’s position gradually, but the movement still feels jerky and unnatural.
I’m wondering if any of you have experience or suggestions on how to achieve this smooth camera tracking effect in Godot. Are there specific techniques or nodes that I should be using?
https://github.com/godotengine/godot-proposals/issues/6389info
Perhaps there are some tutorials or resources you could point me towards that address this issue?
Any advice or insights you could provide would be greatly appreciated.
Thank you in advance for your help.
1 Like
Hi,
I might have miss understood the question. Isn’t the answer just to make the camera a child of the character, therefore its at the same location as the character?
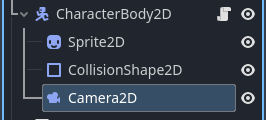
Then just control the character however you like, for instance if you are doing a front facing platformer as opposed to a top down environment you could do stuff like:
extends CharacterBody2D
const SPEED = 150.0
const FLOOR_DRAG = 200.0
const AIR_DRAG = 20.0
const JUMP_DRAG = 10.0
const MAX_JUMP_VELOCITY = -400.0
const JUMP_SPEED = 1000.0
var gravity = ProjectSettings.get_setting("physics/2d/default_gravity")
var jump_buildup = 0
var required_speed = 0
func _physics_process(delta):
var change_in_velocity
if is_on_floor():
# you should replace UI actions with custom gameplay actions.
var direction = Input.get_axis("ui_left", "ui_right")
if direction:
change_in_velocity = SPEED
required_speed = direction * SPEED
else:
change_in_velocity = FLOOR_DRAG
required_speed = 0
# Handle Jump.
if Input.is_action_pressed("ui_accept"):
jump_buildup = move_toward(jump_buildup, MAX_JUMP_VELOCITY, JUMP_SPEED * delta)
elif Input.is_action_just_released("ui_accept"):
velocity.y = jump_buildup
jump_buildup = 0
else:
required_speed = move_toward(required_speed, 0, JUMP_DRAG * delta)
change_in_velocity = AIR_DRAG
# Add the gravity.
velocity.y += gravity * delta
jump_buildup = 0
velocity.x = move_toward(velocity.x, required_speed, change_in_velocity * delta)
move_and_slide()
Apologies if I’ve miss understood the question.
The easiest way is to use Phantom Camera plugin. It has so many features.