Godot Version
4.2 Mono
Question
Im trying to connect to the on_body_entered signal however I can’t seem to figure out what that actually looks like in C#. in GD script when you connect a signal it automagically shows up in the script
this is what i have now just so i could test to make sure collision was working but obviously this wont do for actual gameplay
using Godot;
public partial class LineOfSight : Area2D
{
public override void _Process(double delta)
{
var body = GetOverlappingBodies();
GD.Print(body);
}
if you are using visual studio, type ‘body’ to see auto complete suggestions.
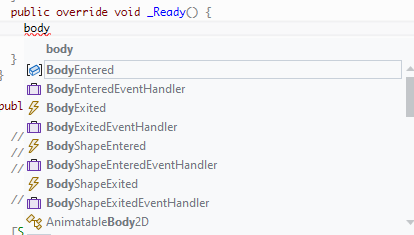
this is how to add your function BodyEntered:
public partial class MyArea:Area2D {
void MyBodyEnteredEventHandler(Node2D body) {
}
public override void _Ready() {
BodyEntered += MyBodyEnteredEventHandler;
}
}
it also helps to examine godot class metadata. in visual studio i ctrl+click Area2D:

it takes some digging but the information you need is in the metadata
public event BodyEnteredEventHandler BodyEntered;
public event BodyExitedEventHandler BodyExited;
public delegate void BodyEnteredEventHandler(Node2D body);
public delegate void BodyExitedEventHandler(Node2D body);
Got it all figured out
using Godot;
public partial class LineOfSight : Area2D
{
private Node2D player;
void MyBodyEventHandler(Node2D body)
{
if (body == player)
{
GD.Print("Player Found!");
}
}
public override void _Ready()
{
BodyEntered += MyBodyEventHandler;
player = GetTree().GetFirstNodeInGroup("Player") as Node2D;
}
}
for future reference, here is how to connect to godot signals in c#.
using Godot;
using System;
public partial class MyTimer:Timer {
public Action m_timeout_func = null;
void DoTimeout() {
GD.Print("normal function");
Disconnect(Timer.SignalName.Timeout, Callable.From(DoTimeout)); // and how to disconnect the signal
}
public override void _Ready() {
Action m_variable_timeout_function = () => {
GD.Print("function stored as a variable");
};
Start(2); // timer triggers every 2 secs
// connect timeout signals
Connect(Timer.SignalName.Timeout, Callable.From(DoTimeout)); // void DoTimeout() {}
Connect(Timer.SignalName.Timeout, Callable.From(m_variable_timeout_function)); // Action m_timeout_func = () => {}
Connect(Timer.SignalName.Timeout, Callable.From(() => {
GD.Print("anonymous function");
}));
}
}